mirror of
https://github.com/nodejs/node.git
synced 2025-05-15 10:01:49 +00:00
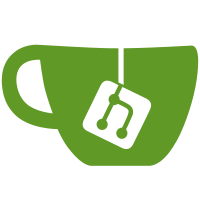
The copyright and license notice is already in the LICENSE file. There is no justifiable reason to also require that it be included in every file, since the individual files are not individually distributed except as part of the entire package.
69 lines
1.6 KiB
JavaScript
69 lines
1.6 KiB
JavaScript
// a duplex stream is just a stream that is both readable and writable.
|
|
// Since JS doesn't have multiple prototypal inheritance, this class
|
|
// prototypally inherits from Readable, and then parasitically from
|
|
// Writable.
|
|
|
|
module.exports = Duplex;
|
|
|
|
/*<replacement>*/
|
|
var objectKeys = Object.keys || function (obj) {
|
|
var keys = [];
|
|
for (var key in obj) keys.push(key);
|
|
return keys;
|
|
}
|
|
/*</replacement>*/
|
|
|
|
|
|
/*<replacement>*/
|
|
var util = require('core-util-is');
|
|
util.inherits = require('inherits');
|
|
/*</replacement>*/
|
|
|
|
var Readable = require('./_stream_readable');
|
|
var Writable = require('./_stream_writable');
|
|
|
|
util.inherits(Duplex, Readable);
|
|
|
|
forEach(objectKeys(Writable.prototype), function(method) {
|
|
if (!Duplex.prototype[method])
|
|
Duplex.prototype[method] = Writable.prototype[method];
|
|
});
|
|
|
|
function Duplex(options) {
|
|
if (!(this instanceof Duplex))
|
|
return new Duplex(options);
|
|
|
|
Readable.call(this, options);
|
|
Writable.call(this, options);
|
|
|
|
if (options && options.readable === false)
|
|
this.readable = false;
|
|
|
|
if (options && options.writable === false)
|
|
this.writable = false;
|
|
|
|
this.allowHalfOpen = true;
|
|
if (options && options.allowHalfOpen === false)
|
|
this.allowHalfOpen = false;
|
|
|
|
this.once('end', onend);
|
|
}
|
|
|
|
// the no-half-open enforcer
|
|
function onend() {
|
|
// if we allow half-open state, or if the writable side ended,
|
|
// then we're ok.
|
|
if (this.allowHalfOpen || this._writableState.ended)
|
|
return;
|
|
|
|
// no more data can be written.
|
|
// But allow more writes to happen in this tick.
|
|
process.nextTick(this.end.bind(this));
|
|
}
|
|
|
|
function forEach (xs, f) {
|
|
for (var i = 0, l = xs.length; i < l; i++) {
|
|
f(xs[i], i);
|
|
}
|
|
}
|