mirror of
https://github.com/nodejs/node.git
synced 2025-05-04 15:02:25 +00:00
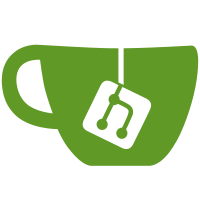
this change makes `deflate-raw` a valid parameter for both CompressionStream and DecompressionStream constructors it makes node's implementation consistent with what modern browsers support and what specification calls for see: https://wicg.github.io/compression/#compression-stream PR-URL: https://github.com/nodejs/node/pull/50097 Reviewed-By: Yagiz Nizipli <yagiz@nizipli.com> Reviewed-By: Tobias Nießen <tniessen@tnie.de> Reviewed-By: Luigi Pinca <luigipinca@gmail.com> Reviewed-By: James M Snell <jasnell@gmail.com> Reviewed-By: Filip Skokan <panva.ip@gmail.com>
143 lines
2.9 KiB
JavaScript
143 lines
2.9 KiB
JavaScript
'use strict';
|
|
|
|
const {
|
|
ObjectDefineProperties,
|
|
} = primordials;
|
|
|
|
const {
|
|
codes: { ERR_INVALID_ARG_VALUE },
|
|
} = require('internal/errors');
|
|
|
|
const {
|
|
newReadableWritablePairFromDuplex,
|
|
} = require('internal/webstreams/adapters');
|
|
|
|
const { customInspect } = require('internal/webstreams/util');
|
|
|
|
const {
|
|
customInspectSymbol: kInspect,
|
|
kEnumerableProperty,
|
|
} = require('internal/util');
|
|
|
|
let zlib;
|
|
function lazyZlib() {
|
|
zlib ??= require('zlib');
|
|
return zlib;
|
|
}
|
|
|
|
/**
|
|
* @typedef {import('./readablestream').ReadableStream} ReadableStream
|
|
* @typedef {import('./writablestream').WritableStream} WritableStream
|
|
*/
|
|
|
|
class CompressionStream {
|
|
#handle;
|
|
#transform;
|
|
|
|
/**
|
|
* @param {'deflate'|'deflate-raw'|'gzip'} format
|
|
*/
|
|
constructor(format) {
|
|
switch (format) {
|
|
case 'deflate':
|
|
this.#handle = lazyZlib().createDeflate();
|
|
break;
|
|
case 'deflate-raw':
|
|
this.#handle = lazyZlib().createDeflateRaw();
|
|
break;
|
|
case 'gzip':
|
|
this.#handle = lazyZlib().createGzip();
|
|
break;
|
|
default:
|
|
throw new ERR_INVALID_ARG_VALUE('format', format);
|
|
}
|
|
this.#transform = newReadableWritablePairFromDuplex(this.#handle);
|
|
}
|
|
|
|
/**
|
|
* @readonly
|
|
* @type {ReadableStream}
|
|
*/
|
|
get readable() {
|
|
return this.#transform.readable;
|
|
}
|
|
|
|
/**
|
|
* @readonly
|
|
* @type {WritableStream}
|
|
*/
|
|
get writable() {
|
|
return this.#transform.writable;
|
|
}
|
|
|
|
[kInspect](depth, options) {
|
|
customInspect(depth, options, 'CompressionStream', {
|
|
readable: this.#transform.readable,
|
|
writable: this.#transform.writable,
|
|
});
|
|
}
|
|
}
|
|
|
|
class DecompressionStream {
|
|
#handle;
|
|
#transform;
|
|
|
|
/**
|
|
* @param {'deflate'|'deflate-raw'|'gzip'} format
|
|
*/
|
|
constructor(format) {
|
|
switch (format) {
|
|
case 'deflate':
|
|
this.#handle = lazyZlib().createInflate();
|
|
break;
|
|
case 'deflate-raw':
|
|
this.#handle = lazyZlib().createInflateRaw();
|
|
break;
|
|
case 'gzip':
|
|
this.#handle = lazyZlib().createGunzip();
|
|
break;
|
|
default:
|
|
throw new ERR_INVALID_ARG_VALUE('format', format);
|
|
}
|
|
this.#transform = newReadableWritablePairFromDuplex(this.#handle);
|
|
}
|
|
|
|
/**
|
|
* @readonly
|
|
* @type {ReadableStream}
|
|
*/
|
|
get readable() {
|
|
return this.#transform.readable;
|
|
}
|
|
|
|
/**
|
|
* @readonly
|
|
* @type {WritableStream}
|
|
*/
|
|
get writable() {
|
|
return this.#transform.writable;
|
|
}
|
|
|
|
[kInspect](depth, options) {
|
|
customInspect(depth, options, 'DecompressionStream', {
|
|
readable: this.#transform.readable,
|
|
writable: this.#transform.writable,
|
|
});
|
|
}
|
|
}
|
|
|
|
ObjectDefineProperties(CompressionStream.prototype, {
|
|
readable: kEnumerableProperty,
|
|
writable: kEnumerableProperty,
|
|
});
|
|
|
|
ObjectDefineProperties(DecompressionStream.prototype, {
|
|
readable: kEnumerableProperty,
|
|
writable: kEnumerableProperty,
|
|
});
|
|
|
|
module.exports = {
|
|
CompressionStream,
|
|
DecompressionStream,
|
|
};
|