mirror of
https://github.com/nodejs/node.git
synced 2025-05-03 00:27:26 +00:00
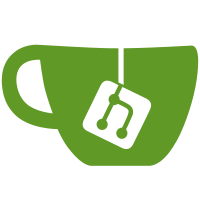
Adds destroy() and _destroy() methods to Readable, Writable, Duplex and Transform. It also standardizes the behavior and the implementation of destroy(), which has been inconsistent in userland and core. This PR also updates all the subsystems of core to use the new destroy(). PR-URL: https://github.com/nodejs/node/pull/12925 Reviewed-By: James M Snell <jasnell@gmail.com> Reviewed-By: Calvin Metcalf <calvin.metcalf@gmail.com> Reviewed-By: Colin Ihrig <cjihrig@gmail.com>
66 lines
1.6 KiB
JavaScript
66 lines
1.6 KiB
JavaScript
'use strict';
|
|
|
|
// undocumented cb() API, needed for core, not for public API
|
|
function destroy(err, cb) {
|
|
const readableDestroyed = this._readableState &&
|
|
this._readableState.destroyed;
|
|
const writableDestroyed = this._writableState &&
|
|
this._writableState.destroyed;
|
|
|
|
if (readableDestroyed || writableDestroyed) {
|
|
if (err && (!this._writableState || !this._writableState.errorEmitted)) {
|
|
process.nextTick(emitErrorNT, this, err);
|
|
}
|
|
return;
|
|
}
|
|
|
|
// we set destroyed to true before firing error callbacks in order
|
|
// to make it re-entrance safe in case destroy() is called within callbacks
|
|
|
|
if (this._readableState) {
|
|
this._readableState.destroyed = true;
|
|
}
|
|
|
|
// if this is a duplex stream mark the writable part as destroyed as well
|
|
if (this._writableState) {
|
|
this._writableState.destroyed = true;
|
|
}
|
|
|
|
this._destroy(err || null, (err) => {
|
|
if (!cb && err) {
|
|
process.nextTick(emitErrorNT, this, err);
|
|
if (this._writableState) {
|
|
this._writableState.errorEmitted = true;
|
|
}
|
|
} else if (cb) {
|
|
cb(err);
|
|
}
|
|
});
|
|
}
|
|
|
|
function undestroy() {
|
|
if (this._readableState) {
|
|
this._readableState.destroyed = false;
|
|
this._readableState.reading = false;
|
|
this._readableState.ended = false;
|
|
this._readableState.endEmitted = false;
|
|
}
|
|
|
|
if (this._writableState) {
|
|
this._writableState.destroyed = false;
|
|
this._writableState.ended = false;
|
|
this._writableState.ending = false;
|
|
this._writableState.finished = false;
|
|
this._writableState.errorEmitted = false;
|
|
}
|
|
}
|
|
|
|
function emitErrorNT(self, err) {
|
|
self.emit('error', err);
|
|
}
|
|
|
|
module.exports = {
|
|
destroy,
|
|
undestroy
|
|
};
|