mirror of
https://github.com/nodejs/node.git
synced 2025-05-04 14:26:57 +00:00
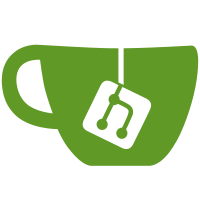
Limit the number of messages processed without interruption on a given `MessagePort` to prevent event loop starvation, but still make sure that all messages are emitted that were already in the queue when emitting began. This aligns the behaviour better with the web. Refs: https://github.com/nodejs/node/pull/28030 PR-URL: https://github.com/nodejs/node/pull/29315 Reviewed-By: Gus Caplan <me@gus.host> Reviewed-By: Colin Ihrig <cjihrig@gmail.com> Reviewed-By: James M Snell <jasnell@gmail.com> Reviewed-By: Jeremiah Senkpiel <fishrock123@rocketmail.com>
30 lines
869 B
JavaScript
30 lines
869 B
JavaScript
'use strict';
|
|
const common = require('../common');
|
|
const assert = require('assert');
|
|
|
|
const { MessageChannel } = require('worker_threads');
|
|
|
|
// Make sure that an infinite asynchronous .on('message')/postMessage loop
|
|
// does not lead to a stack overflow and does not starve the event loop.
|
|
// We schedule timeouts both from before the the .on('message') handler and
|
|
// inside of it, which both should run.
|
|
|
|
const { port1, port2 } = new MessageChannel();
|
|
let count = 0;
|
|
port1.on('message', () => {
|
|
if (count === 0) {
|
|
setTimeout(common.mustCall(() => {
|
|
port1.close();
|
|
}), 0);
|
|
}
|
|
|
|
port2.postMessage(0);
|
|
assert(count++ < 10000, `hit ${count} loop iterations`);
|
|
});
|
|
|
|
port2.postMessage(0);
|
|
|
|
// This is part of the test -- the event loop should be available and not stall
|
|
// out due to the recursive .postMessage() calls.
|
|
setTimeout(common.mustCall(), 0);
|