mirror of
https://github.com/nodejs/node.git
synced 2025-05-03 09:52:21 +00:00
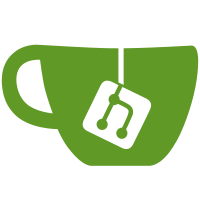
The TLS trace data is best-effort, and enough can be dropped from pipe buffers that only the start of the trace is detected. Only assert on the first line of the trace, it should not get dropped, and it's enough to check that trace was enabled via CLI. PR-URL: https://github.com/nodejs/node/pull/28043 Fixes: https://github.com/nodejs/node/issues/27636 Reviewed-By: Luigi Pinca <luigipinca@gmail.com> Reviewed-By: Colin Ihrig <cjihrig@gmail.com> Reviewed-By: Richard Lau <riclau@uk.ibm.com> Reviewed-By: Ruben Bridgewater <ruben@bridgewater.de>
69 lines
1.8 KiB
JavaScript
69 lines
1.8 KiB
JavaScript
// Flags: --expose-internals
|
|
'use strict';
|
|
const common = require('../common');
|
|
if (!common.hasCrypto) common.skip('missing crypto');
|
|
const fixtures = require('../common/fixtures');
|
|
|
|
// Test --trace-tls CLI flag.
|
|
|
|
const assert = require('assert');
|
|
const { fork } = require('child_process');
|
|
|
|
if (process.argv[2] === 'test')
|
|
return test();
|
|
|
|
const binding = require('internal/test/binding').internalBinding;
|
|
|
|
if (!binding('tls_wrap').HAVE_SSL_TRACE)
|
|
return common.skip('no SSL_trace() compiled into openssl');
|
|
|
|
const child = fork(__filename, ['test'], {
|
|
silent: true,
|
|
execArgv: ['--trace-tls']
|
|
});
|
|
|
|
let stdout = '';
|
|
let stderr = '';
|
|
child.stdout.setEncoding('utf8');
|
|
child.stderr.setEncoding('utf8');
|
|
child.stdout.on('data', (data) => stdout += data);
|
|
child.stderr.on('data', (data) => stderr += data);
|
|
child.on('close', common.mustCall((code, signal) => {
|
|
// For debugging and observation of actual trace output.
|
|
console.log(stderr);
|
|
|
|
assert.strictEqual(code, 0);
|
|
assert.strictEqual(signal, null);
|
|
assert.strictEqual(stdout.trim(), '');
|
|
assert(/Warning: Enabling --trace-tls can expose sensitive/.test(stderr));
|
|
assert(/Sent Record/.test(stderr));
|
|
}));
|
|
|
|
function test() {
|
|
const {
|
|
connect, keys
|
|
} = require(fixtures.path('tls-connect'));
|
|
|
|
connect({
|
|
client: {
|
|
checkServerIdentity: (servername, cert) => { },
|
|
ca: `${keys.agent1.cert}\n${keys.agent6.ca}`,
|
|
},
|
|
server: {
|
|
cert: keys.agent6.cert,
|
|
key: keys.agent6.key
|
|
},
|
|
}, common.mustCall((err, pair, cleanup) => {
|
|
if (pair.server.err) {
|
|
console.trace('server', pair.server.err);
|
|
}
|
|
if (pair.client.err) {
|
|
console.trace('client', pair.client.err);
|
|
}
|
|
assert.ifError(pair.server.err);
|
|
assert.ifError(pair.client.err);
|
|
|
|
return cleanup();
|
|
}));
|
|
}
|