mirror of
https://github.com/nodejs/node.git
synced 2025-05-03 13:28:42 +00:00
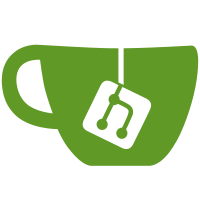
Add capabilities to common test module to detect and skip tests on dumb terminals. In some of our build environments, like s390x, the terminal is a dumb terminal meaning it has very rudimentary capabilities. These in turn prevent some of the tests from completing with errors as below. not ok 1777 parallel/test-readline-tab-complete --- duration_ms: 0.365 severity: fail exitcode: 1 stack: |- assert.js:103 throw new AssertionError(obj); ^ AssertionError [ERR_ASSERTION]: Expected values to be strictly equal: '\t' !== '' at /home/abuild/rpmbuild/BUILD/node-git.8698dd98bb/test/parallel/test-readline-tab-complete.js:63:14 at Array.forEach (<anonymous>) at /home/abuild/rpmbuild/BUILD/node-git.8698dd98bb/test/parallel/test-readline-tab-complete.js:18:17 at Array.forEach (<anonymous>) at Object.<anonymous> (/home/abuild/rpmbuild/BUILD/node-git.8698dd98bb/test/parallel/test-readline-tab-complete.js:17:3) at Module._compile (internal/modules/cjs/loader.js:1176:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:1196:10) at Module.load (internal/modules/cjs/loader.js:1040:32) at Function.Module._load (internal/modules/cjs/loader.js:929:14) at Function.executeUserEntryPoint [as runMain] (internal/modules/run_main.js:71:12) { generatedMessage: true, code: 'ERR_ASSERTION', actual: '\t', expected: '', operator: 'strictEqual' } ... PR-URL: https://github.com/nodejs/node/pull/33165 Reviewed-By: Ruben Bridgewater <ruben@bridgewater.de> Reviewed-By: Anna Henningsen <anna@addaleax.net>
45 lines
1.0 KiB
JavaScript
45 lines
1.0 KiB
JavaScript
'use strict';
|
||
|
||
const common = require('../common');
|
||
const assert = require('assert');
|
||
const PassThrough = require('stream').PassThrough;
|
||
const readline = require('readline');
|
||
|
||
common.skipIfDumbTerminal();
|
||
|
||
// Checks that tab completion still works
|
||
// when output column size is undefined
|
||
|
||
const iStream = new PassThrough();
|
||
const oStream = new PassThrough();
|
||
|
||
readline.createInterface({
|
||
terminal: true,
|
||
input: iStream,
|
||
output: oStream,
|
||
completer: function(line, cb) {
|
||
cb(null, [['process.stdout', 'process.stdin', 'process.stderr'], line]);
|
||
}
|
||
});
|
||
|
||
let output = '';
|
||
|
||
oStream.on('data', function(data) {
|
||
output += data;
|
||
});
|
||
|
||
oStream.on('end', common.mustCall(() => {
|
||
const expect = 'process.stdout\r\n' +
|
||
'process.stdin\r\n' +
|
||
'process.stderr';
|
||
assert(new RegExp(expect).test(output));
|
||
}));
|
||
|
||
iStream.write('process.s\t');
|
||
|
||
assert(/process\.std\b/.test(output)); // Completion works.
|
||
assert(!/stdout/.test(output)); // Completion doesn’t show all results yet.
|
||
|
||
iStream.write('\t');
|
||
oStream.end();
|