mirror of
https://github.com/nodejs/node.git
synced 2025-05-03 09:52:21 +00:00
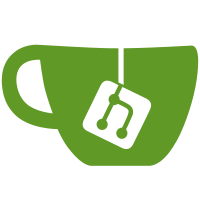
Adds a new `perf_hooks.createHistogram()` API for creating histogram instances that allow user recording. Makes Histogram instances cloneable via MessagePort. This allows, for instance, an event loop delay monitor to be running on the main thread while the histogram data can be monitored actively from a worker thread. Signed-off-by: James M Snell <jasnell@gmail.com> PR-URL: https://github.com/nodejs/node/pull/37155 Reviewed-By: Matteo Collina <matteo.collina@gmail.com>
70 lines
1.7 KiB
JavaScript
70 lines
1.7 KiB
JavaScript
'use strict';
|
|
|
|
const common = require('../common');
|
|
const assert = require('assert');
|
|
const {
|
|
createHistogram,
|
|
monitorEventLoopDelay,
|
|
} = require('perf_hooks');
|
|
|
|
{
|
|
const h = createHistogram();
|
|
|
|
assert.strictEqual(h.min, 9223372036854776000);
|
|
assert.strictEqual(h.max, 0);
|
|
assert.strictEqual(h.exceeds, 0);
|
|
assert(Number.isNaN(h.mean));
|
|
assert(Number.isNaN(h.stddev));
|
|
|
|
h.record(1);
|
|
|
|
[false, '', {}, undefined, null].forEach((i) => {
|
|
assert.throws(() => h.record(i), {
|
|
code: 'ERR_INVALID_ARG_TYPE'
|
|
});
|
|
});
|
|
assert.throws(() => h.record(0, Number.MAX_SAFE_INTEGER + 1), {
|
|
code: 'ERR_OUT_OF_RANGE'
|
|
});
|
|
|
|
assert.strictEqual(h.min, 1);
|
|
assert.strictEqual(h.max, 1);
|
|
assert.strictEqual(h.exceeds, 0);
|
|
assert.strictEqual(h.mean, 1);
|
|
assert.strictEqual(h.stddev, 0);
|
|
|
|
assert.strictEqual(h.percentile(1), 1);
|
|
assert.strictEqual(h.percentile(100), 1);
|
|
|
|
const mc = new MessageChannel();
|
|
mc.port1.onmessage = common.mustCall(({ data }) => {
|
|
assert.strictEqual(h.min, 1);
|
|
assert.strictEqual(h.max, 1);
|
|
assert.strictEqual(h.exceeds, 0);
|
|
assert.strictEqual(h.mean, 1);
|
|
assert.strictEqual(h.stddev, 0);
|
|
|
|
data.record(2n);
|
|
data.recordDelta();
|
|
|
|
assert.strictEqual(h.max, 2);
|
|
|
|
mc.port1.close();
|
|
});
|
|
mc.port2.postMessage(h);
|
|
}
|
|
|
|
{
|
|
const e = monitorEventLoopDelay();
|
|
e.enable();
|
|
const mc = new MessageChannel();
|
|
mc.port1.onmessage = common.mustCall(({ data }) => {
|
|
assert(typeof data.min, 'number');
|
|
assert(data.min > 0);
|
|
assert.strictEqual(data.disable, undefined);
|
|
assert.strictEqual(data.enable, undefined);
|
|
mc.port1.close();
|
|
});
|
|
setTimeout(() => mc.port2.postMessage(e), 100);
|
|
}
|