mirror of
https://github.com/nodejs/node.git
synced 2025-05-03 13:28:42 +00:00
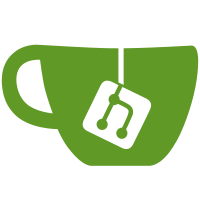
Remove magic numbers (500, 10, 100) from the test. Instead, detect when GC has started and stop sending requests at that point. On my laptop, this results in 16 or 20 requests per run instead of 500. Fixes: https://github.com/nodejs/node/issues/23089 PR-URL: https://github.com/nodejs/node/pull/24943 Reviewed-By: Colin Ihrig <cjihrig@gmail.com>
73 lines
1.3 KiB
JavaScript
73 lines
1.3 KiB
JavaScript
'use strict';
|
|
// Flags: --expose-gc
|
|
// just like test-gc-http-client.js,
|
|
// but with an on('error') handler that does nothing.
|
|
|
|
const common = require('../common');
|
|
const onGC = require('../common/ongc');
|
|
|
|
const cpus = require('os').cpus().length;
|
|
|
|
function serverHandler(req, res) {
|
|
req.resume();
|
|
res.writeHead(200, { 'Content-Type': 'text/plain' });
|
|
res.end('Hello World\n');
|
|
}
|
|
|
|
const http = require('http');
|
|
let createClients = true;
|
|
let done = 0;
|
|
let count = 0;
|
|
let countGC = 0;
|
|
|
|
const server = http.createServer(serverHandler);
|
|
server.listen(0, common.mustCall(() => {
|
|
for (let i = 0; i < cpus; i++)
|
|
getAll();
|
|
}));
|
|
|
|
function getAll() {
|
|
if (createClients) {
|
|
const req = http.get({
|
|
hostname: 'localhost',
|
|
pathname: '/',
|
|
port: server.address().port
|
|
}, cb).on('error', onerror);
|
|
|
|
count++;
|
|
onGC(req, { ongc });
|
|
|
|
setImmediate(getAll);
|
|
}
|
|
}
|
|
|
|
function cb(res) {
|
|
res.resume();
|
|
done++;
|
|
}
|
|
|
|
function onerror(err) {
|
|
throw err;
|
|
}
|
|
|
|
function ongc() {
|
|
countGC++;
|
|
}
|
|
|
|
setImmediate(status);
|
|
|
|
function status() {
|
|
if (done > 0) {
|
|
createClients = false;
|
|
global.gc();
|
|
console.log(`done/collected/total: ${done}/${countGC}/${count}`);
|
|
if (countGC === count) {
|
|
server.close();
|
|
} else {
|
|
setImmediate(status);
|
|
}
|
|
} else {
|
|
setImmediate(status);
|
|
}
|
|
}
|