mirror of
https://github.com/nodejs/node.git
synced 2025-05-07 12:03:30 +00:00
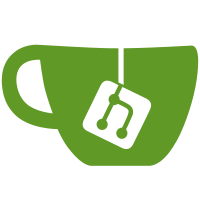
Keep the `ArrayBuffer::Allocator` behind a `SharedArrayBuffer` instance alive for at least as long as the receiving Isolate lives, if the `SharedArrayBuffer` instance isn’t already destroyed through GC. This is to work around the fact that V8 7.9 started refactoring how backing stores for `SharedArrayBuffer` instances work, changing the timing of the call that releases the backing store to be during Isolate disposal. The flag added to the test is optional but helps verify that the backing store is actually free’d at the end of the test and does not leak memory. Fixes: https://github.com/nodejs/node-v8/issues/115 PR-URL: https://github.com/nodejs/node/pull/29637 Reviewed-By: Rich Trott <rtrott@gmail.com> Reviewed-By: Michaël Zasso <targos@protonmail.com> Reviewed-By: Ben Noordhuis <info@bnoordhuis.nl>
24 lines
837 B
JavaScript
24 lines
837 B
JavaScript
// Flags: --debug-arraybuffer-allocations
|
|
'use strict';
|
|
const common = require('../common');
|
|
const assert = require('assert');
|
|
const { Worker } = require('worker_threads');
|
|
|
|
// Regression test for https://github.com/nodejs/node/issues/28777
|
|
// Make sure that SharedArrayBuffers created in Worker threads are accessible
|
|
// after the creating thread ended.
|
|
|
|
const w = new Worker(`
|
|
const { parentPort } = require('worker_threads');
|
|
const sharedArrayBuffer = new SharedArrayBuffer(4);
|
|
parentPort.postMessage(sharedArrayBuffer);
|
|
`, { eval: true });
|
|
|
|
let sharedArrayBuffer;
|
|
w.once('message', common.mustCall((message) => sharedArrayBuffer = message));
|
|
w.once('exit', common.mustCall(() => {
|
|
const uint8array = new Uint8Array(sharedArrayBuffer);
|
|
uint8array[0] = 42;
|
|
assert.deepStrictEqual(uint8array, new Uint8Array([42, 0, 0, 0]));
|
|
}));
|