mirror of
https://github.com/nodejs/node.git
synced 2025-05-07 15:35:41 +00:00
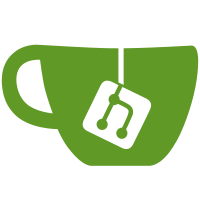
If a readable stream was set up with `highWaterMark 0`, the while-loop in `maybeReadMore_` function would never execute. The while loop now has an extra or-condition for the case where the stream is flowing and there are no items. The or-condition is adapted from the emit-condition of the `addChunk` function. The `addChunk` also contains a check for `state.sync`. However that part of the check was omitted here because the `maybeReadMore_` is executed using `process.nextTick`. `state.sync` is set and then unset within the `read()` function so it should never be in effect in `maybeReadMore_`. Fixes: https://github.com/nodejs/node/issues/24915 PR-URL: https://github.com/nodejs/node/pull/24918 Reviewed-By: Anna Henningsen <anna@addaleax.net> Reviewed-By: Matteo Collina <matteo.collina@gmail.com>
28 lines
645 B
JavaScript
28 lines
645 B
JavaScript
'use strict';
|
|
|
|
const common = require('../common');
|
|
|
|
// This test ensures that Readable stream will continue to call _read
|
|
// for streams with highWaterMark === 0 once the stream returns data
|
|
// by calling push() asynchronously.
|
|
|
|
const { Readable } = require('stream');
|
|
|
|
let count = 5;
|
|
|
|
const r = new Readable({
|
|
// Called 6 times: First 5 return data, last one signals end of stream.
|
|
read: common.mustCall(() => {
|
|
process.nextTick(common.mustCall(() => {
|
|
if (count--)
|
|
r.push('a');
|
|
else
|
|
r.push(null);
|
|
}));
|
|
}, 6),
|
|
highWaterMark: 0,
|
|
});
|
|
|
|
r.on('end', common.mustCall());
|
|
r.on('data', common.mustCall(5));
|