mirror of
https://github.com/nodejs/node.git
synced 2025-05-07 17:32:22 +00:00
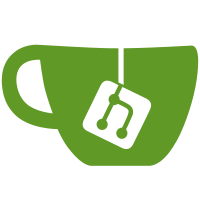
This makes sure that the described default behavior for the `terminal` option is actually always used and not only when running the REPL as standalone program. The options code is now logically combined instead of being spread out in the big REPL constructor. PR-URL: https://github.com/nodejs/node/pull/26518 Reviewed-By: Lance Ball <lball@redhat.com> Reviewed-By: Matteo Collina <matteo.collina@gmail.com> Reviewed-By: Rich Trott <rtrott@gmail.com> Reviewed-By: James M Snell <jasnell@gmail.com>
35 lines
1.1 KiB
JavaScript
35 lines
1.1 KiB
JavaScript
/* eslint-disable quotes */
|
|
'use strict';
|
|
require('../common');
|
|
const { Duplex } = require('stream');
|
|
const { inspect } = require('util');
|
|
const { strictEqual } = require('assert');
|
|
const { REPLServer } = require('repl');
|
|
|
|
let output = '';
|
|
|
|
const inout = new Duplex({ decodeStrings: false });
|
|
inout._read = function() {
|
|
this.push('util.inspect("string")\n');
|
|
this.push(null);
|
|
};
|
|
inout._write = function(s, _, cb) {
|
|
output += s;
|
|
cb();
|
|
};
|
|
|
|
const repl = new REPLServer({ input: inout, output: inout, useColors: true });
|
|
inout.isTTY = true;
|
|
const repl2 = new REPLServer({ input: inout, output: inout });
|
|
|
|
process.on('exit', function() {
|
|
// https://github.com/nodejs/node/pull/16485#issuecomment-350428638
|
|
// The color setting of the REPL should not have leaked over into
|
|
// the color setting of `util.inspect.defaultOptions`.
|
|
strictEqual(output.includes(`"'string'"`), true);
|
|
strictEqual(output.includes(`'\u001b[32m\\'string\\'\u001b[39m'`), false);
|
|
strictEqual(inspect.defaultOptions.colors, false);
|
|
strictEqual(repl.writer.options.colors, true);
|
|
strictEqual(repl2.writer.options.colors, true);
|
|
});
|