mirror of
https://github.com/nodejs/node.git
synced 2025-04-30 15:41:06 +00:00
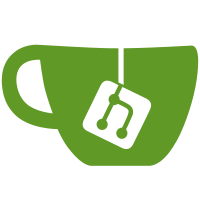
common.js needs to be loaded in all tests so that there is checking for variable leaks and possibly other things. However, it does not need to be assigned to a variable if nothing in common.js is referred to elsewhere in the test. PR-URL: https://github.com/nodejs/node/pull/4408 Reviewed-By: James M Snell <jasnell@gmail.com>
28 lines
801 B
JavaScript
28 lines
801 B
JavaScript
'use strict';
|
|
require('../common');
|
|
var assert = require('assert');
|
|
|
|
var zero = [];
|
|
var one = [ new Buffer('asdf') ];
|
|
var long = [];
|
|
for (var i = 0; i < 10; i++) long.push(new Buffer('asdf'));
|
|
|
|
var flatZero = Buffer.concat(zero);
|
|
var flatOne = Buffer.concat(one);
|
|
var flatLong = Buffer.concat(long);
|
|
var flatLongLen = Buffer.concat(long, 40);
|
|
|
|
assert(flatZero.length === 0);
|
|
assert(flatOne.toString() === 'asdf');
|
|
// A special case where concat used to return the first item,
|
|
// if the length is one. This check is to make sure that we don't do that.
|
|
assert(flatOne !== one[0]);
|
|
assert(flatLong.toString() === (new Array(10 + 1).join('asdf')));
|
|
assert(flatLongLen.toString() === (new Array(10 + 1).join('asdf')));
|
|
|
|
assert.throws(function() {
|
|
Buffer.concat([42]);
|
|
}, TypeError);
|
|
|
|
console.log('ok');
|