mirror of
https://github.com/nodejs/node.git
synced 2025-05-02 13:11:36 +00:00
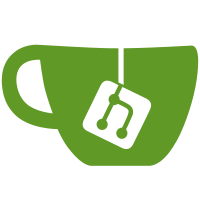
Some checks failed
Coverage Linux (without intl) / coverage-linux-without-intl (push) Waiting to run
Coverage Linux / coverage-linux (push) Waiting to run
Coverage Windows / coverage-windows (push) Waiting to run
Test and upload documentation to artifacts / build-docs (push) Waiting to run
Linters / lint-addon-docs (push) Waiting to run
Linters / lint-cpp (push) Waiting to run
Linters / format-cpp (push) Waiting to run
Linters / lint-js-and-md (push) Waiting to run
Linters / lint-py (push) Waiting to run
Linters / lint-yaml (push) Waiting to run
Linters / lint-sh (push) Waiting to run
Linters / lint-codeowners (push) Waiting to run
Linters / lint-pr-url (push) Waiting to run
Linters / lint-readme (push) Waiting to run
Notify on Push / Notify on Force Push on `main` (push) Waiting to run
Notify on Push / Notify on Push on `main` that lacks metadata (push) Waiting to run
Scorecard supply-chain security / Scorecard analysis (push) Has been cancelled
Find inactive TSC voting members / find (push) Has been cancelled
Signed-off-by: James M Snell <jasnell@gmail.com> PR-URL: https://github.com/nodejs/node/pull/56328 Reviewed-By: Yagiz Nizipli <yagiz@nizipli.com>
83 lines
2.3 KiB
JavaScript
83 lines
2.3 KiB
JavaScript
// Flags: --experimental-quic --no-warnings
|
|
'use strict';
|
|
|
|
const { hasQuic } = require('../common');
|
|
const { Buffer } = require('node:buffer');
|
|
|
|
const {
|
|
describe,
|
|
it,
|
|
} = require('node:test');
|
|
|
|
// TODO(@jasnell): Temporarily skip the test on mac until we can figure
|
|
// out while it is failing on macs in CI but running locally on macs ok.
|
|
const isMac = process.platform === 'darwin';
|
|
const skip = isMac || !hasQuic;
|
|
|
|
async function readAll(readable, resolve) {
|
|
const chunks = [];
|
|
for await (const chunk of readable) {
|
|
chunks.push(chunk);
|
|
}
|
|
resolve(Buffer.concat(chunks));
|
|
}
|
|
|
|
describe('quic basic server/client handshake works', { skip }, async () => {
|
|
const { createPrivateKey } = require('node:crypto');
|
|
const fixtures = require('../common/fixtures');
|
|
const keys = createPrivateKey(fixtures.readKey('agent1-key.pem'));
|
|
const certs = fixtures.readKey('agent1-cert.pem');
|
|
|
|
const {
|
|
listen,
|
|
connect,
|
|
} = require('node:quic');
|
|
|
|
const {
|
|
strictEqual,
|
|
ok,
|
|
} = require('node:assert');
|
|
|
|
it('a quic client can connect to a quic server in the same process', async () => {
|
|
const p1 = Promise.withResolvers();
|
|
const p2 = Promise.withResolvers();
|
|
const p3 = Promise.withResolvers();
|
|
|
|
const serverEndpoint = await listen((serverSession) => {
|
|
|
|
serverSession.opened.then((info) => {
|
|
strictEqual(info.servername, 'localhost');
|
|
strictEqual(info.protocol, 'h3');
|
|
strictEqual(info.cipher, 'TLS_AES_128_GCM_SHA256');
|
|
p1.resolve();
|
|
});
|
|
|
|
serverSession.onstream = (stream) => {
|
|
readAll(stream.readable, p3.resolve).then(() => {
|
|
serverSession.close();
|
|
});
|
|
};
|
|
}, { keys, certs });
|
|
|
|
ok(serverEndpoint.address !== undefined);
|
|
|
|
const clientSession = await connect(serverEndpoint.address);
|
|
clientSession.opened.then((info) => {
|
|
strictEqual(info.servername, 'localhost');
|
|
strictEqual(info.protocol, 'h3');
|
|
strictEqual(info.cipher, 'TLS_AES_128_GCM_SHA256');
|
|
p2.resolve();
|
|
});
|
|
|
|
const body = new Blob(['hello']);
|
|
const stream = await clientSession.createUnidirectionalStream({
|
|
body,
|
|
});
|
|
ok(stream);
|
|
|
|
const { 2: data } = await Promise.all([p1.promise, p2.promise, p3.promise]);
|
|
clientSession.close();
|
|
strictEqual(Buffer.from(data).toString(), 'hello');
|
|
});
|
|
});
|