mirror of
https://github.com/nodejs/node.git
synced 2025-04-28 13:40:37 +00:00
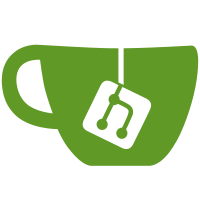
Since `common/crypto` already exists, it makes sense to keep crypto-related utilities there. The only exception being common.hasCrypto which is needed up front to determine if tests should be skipped. Eliminate the redundant check in hasFipsCrypto and just use crypto.getFips() directly where needed. PR-URL: https://github.com/nodejs/node/pull/56714 Reviewed-By: Yagiz Nizipli <yagiz@nizipli.com> Reviewed-By: Luigi Pinca <luigipinca@gmail.com>
55 lines
1.5 KiB
JavaScript
55 lines
1.5 KiB
JavaScript
'use strict';
|
|
|
|
const common = require('../common');
|
|
if (!common.hasCrypto)
|
|
common.skip('missing crypto');
|
|
|
|
const assert = require('assert');
|
|
const {
|
|
createPrivateKey,
|
|
generateKeyPair,
|
|
} = require('crypto');
|
|
const {
|
|
testSignVerify,
|
|
hasOpenSSL3,
|
|
} = require('../common/crypto');
|
|
|
|
// Passing an empty passphrase string should not cause OpenSSL's default
|
|
// passphrase prompt in the terminal.
|
|
// See https://github.com/nodejs/node/issues/35898.
|
|
for (const type of ['pkcs1', 'pkcs8']) {
|
|
generateKeyPair('rsa', {
|
|
modulusLength: 1024,
|
|
privateKeyEncoding: {
|
|
type,
|
|
format: 'pem',
|
|
cipher: 'aes-256-cbc',
|
|
passphrase: ''
|
|
}
|
|
}, common.mustSucceed((publicKey, privateKey) => {
|
|
assert.strictEqual(publicKey.type, 'public');
|
|
|
|
for (const passphrase of ['', Buffer.alloc(0)]) {
|
|
const privateKeyObject = createPrivateKey({
|
|
passphrase,
|
|
key: privateKey
|
|
});
|
|
assert.strictEqual(privateKeyObject.asymmetricKeyType, 'rsa');
|
|
}
|
|
|
|
// Encrypting with an empty passphrase is not the same as not encrypting
|
|
// the key, and not specifying a passphrase should fail when decoding it.
|
|
assert.throws(() => {
|
|
return testSignVerify(publicKey, privateKey);
|
|
}, hasOpenSSL3 ? {
|
|
name: 'Error',
|
|
code: 'ERR_OSSL_CRYPTO_INTERRUPTED_OR_CANCELLED',
|
|
message: 'error:07880109:common libcrypto routines::interrupted or cancelled'
|
|
} : {
|
|
name: 'TypeError',
|
|
code: 'ERR_MISSING_PASSPHRASE',
|
|
message: 'Passphrase required for encrypted key'
|
|
});
|
|
}));
|
|
}
|