mirror of
https://github.com/nodejs/node.git
synced 2025-04-28 13:40:37 +00:00
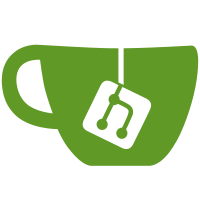
PR-URL: https://github.com/nodejs/node/pull/55698 Reviewed-By: Geoffrey Booth <webadmin@geoffreybooth.com> Reviewed-By: Chengzhong Wu <legendecas@gmail.com> Reviewed-By: Guy Bedford <guybedford@gmail.com>
51 lines
1.5 KiB
JavaScript
51 lines
1.5 KiB
JavaScript
'use strict';
|
|
|
|
require('../common');
|
|
const assert = require('assert');
|
|
const { registerHooks } = require('module');
|
|
|
|
// This tests that the source in the load hook can be returned as
|
|
// array buffers or array buffer views.
|
|
const arrayBufferSource = 'module.exports = "arrayBuffer"';
|
|
const arrayBufferViewSource = 'module.exports = "arrayBufferView"';
|
|
|
|
const encoder = new TextEncoder();
|
|
|
|
const hook1 = registerHooks({
|
|
resolve(specifier, context, nextResolve) {
|
|
return { shortCircuit: true, url: `test://${specifier}` };
|
|
},
|
|
load(url, context, nextLoad) {
|
|
const result = nextLoad(url, context);
|
|
if (url === 'test://array_buffer') {
|
|
assert.deepStrictEqual(result.source, encoder.encode(arrayBufferSource).buffer);
|
|
} else if (url === 'test://array_buffer_view') {
|
|
assert.deepStrictEqual(result.source, encoder.encode(arrayBufferViewSource));
|
|
}
|
|
return result;
|
|
},
|
|
});
|
|
|
|
const hook2 = registerHooks({
|
|
load(url, context, nextLoad) {
|
|
if (url === 'test://array_buffer') {
|
|
return {
|
|
shortCircuit: true,
|
|
source: encoder.encode(arrayBufferSource).buffer,
|
|
};
|
|
} else if (url === 'test://array_buffer_view') {
|
|
return {
|
|
shortCircuit: true,
|
|
source: encoder.encode(arrayBufferViewSource),
|
|
};
|
|
}
|
|
assert.fail('unreachable');
|
|
},
|
|
});
|
|
|
|
assert.strictEqual(require('array_buffer'), 'arrayBuffer');
|
|
assert.strictEqual(require('array_buffer_view'), 'arrayBufferView');
|
|
|
|
hook1.deregister();
|
|
hook2.deregister();
|